Daily Dispatch #5 - Dependency Injection
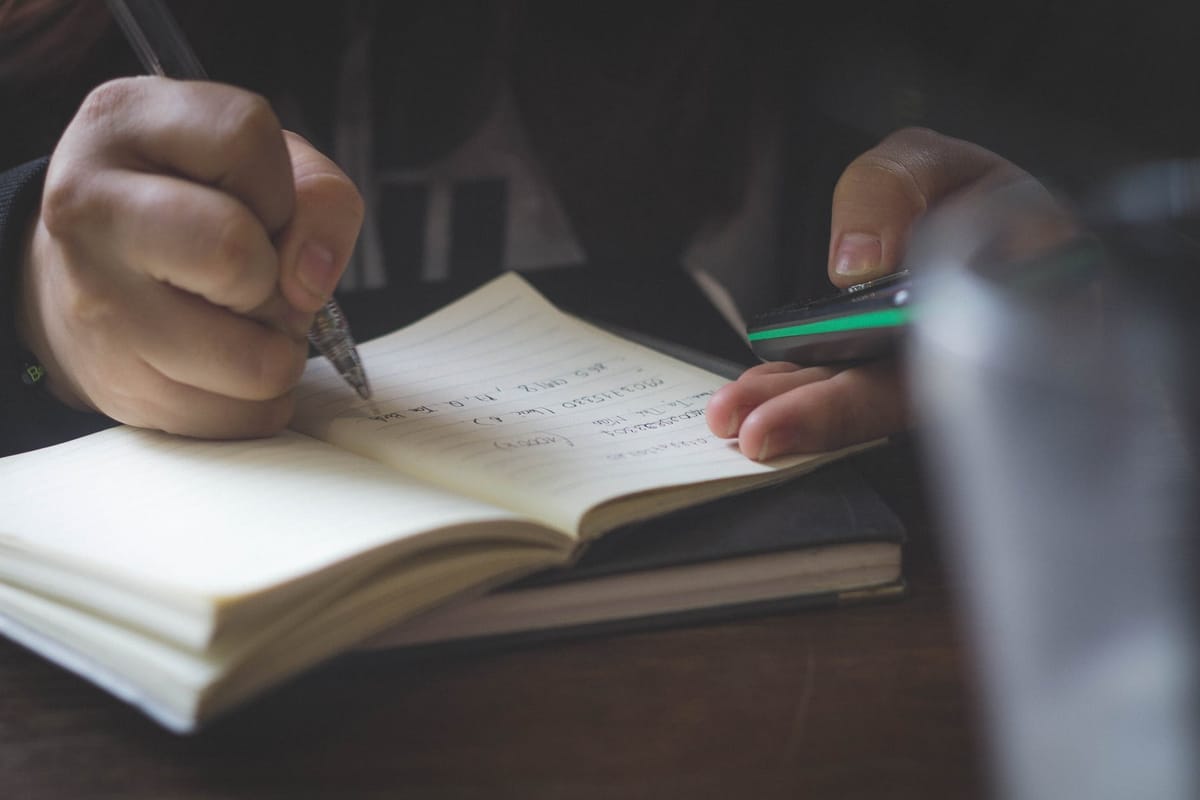
Classes shouldn’t have to fetch their dependencies, you should get a third party module to link implementations with it’s respective interfaces. That way you can change easily the implementations if need be.
Two libraries used for dependency injection are Koin(7.3k stars on GitHub as the time of this post) and Kodein-DI(2.7k stars on GitHub as the time of this post). The main difference that I see is that Kodein-DI works for multi platform apps on Kotlin, however both share many similarities and if your code is neat, you can replace one for the other.
With Koin here’s how it works.
Declaring component’s definitions
// Use the Koin DSL to describe your components definitions // Given some classes class Controller(val service: BusinessService) class BusinessService() // just declare it val myModule = module { single { Controller(get()) } single { BusinessService() } }
Injecting Constructors
// Instances are resolved from your modules // Controller & BusinessService are declared in a module class Controller(val service: BusinessService) { fun hello() { // service is ready to use service.sayHello() } }
Start koin
//Just run startKoin function in your application fun main(vararg args: String) { // start Koin! startKoin { // declare modules modules(myModule) } }
Injecting
// Just inject in a simple Activity class MyActivity() : AppCompatActivity() { // inject BusinessService into property val service: BusinessService by inject() }
Now for Kodein-DI , I’m still trying to make it work.